利用python抓取分析数据包并存入mysql
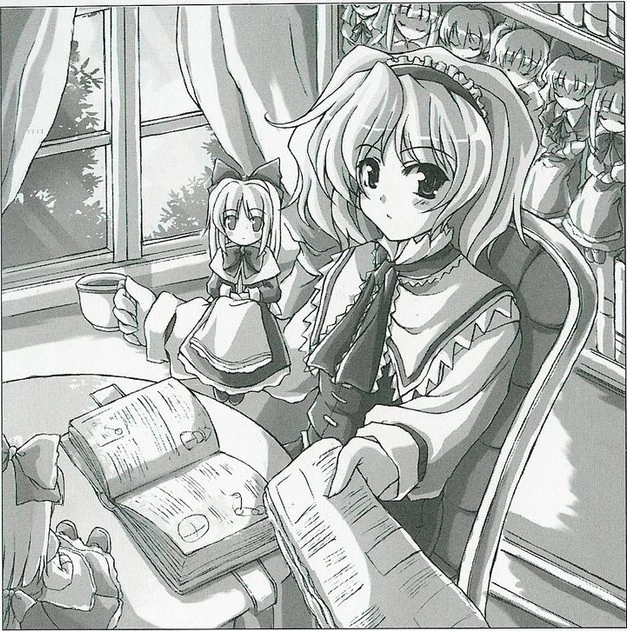
1、先决条件
本机配置好python及mysql运行环境,并且安装好python相关模块scapy
pymysql
2、抓包及分析
抓包功能使用的是scapy中的sniff()函数,使用方法已经记录在前篇: scapy小记pt.4-嗅探 ,本文一定程度上算后篇
3、建立数据库及表
登陆数据库后,建立并指定相关数据库后,建立一张表,提前规划好数据格式
1 | CREATE DATABASE testsql; |
name | dataType |
---|---|
index | u_int() AUTO_INCREMENT |
pktType | char(5) |
capTime | datetime |
srcIP | char(16) |
dstIP | char(16) |
domain | varchar(128) |
4、整体代码
database.py
1 | import pymysql.cursors |
sniffResults.py
1 | from scapy.all import * |
main.py
1 | import database |
参考
使用python抓包并分析后存入数据库,或直接分析tcpdump和wireshark抓到的包,并存入数据库 - 花柒博客-原创分享
- 标题: 利用python抓取分析数据包并存入mysql
- 作者: 7cmb
- 创建于 : 2023-11-30 17:06:36
- 更新于 : 2024-10-12 23:07:35
- 链接: https://7cmb.com/利用python抓取分析数据包并存入mysql/
- 版权声明: 本文章采用 CC BY-NC-SA 4.0 进行许可。